Some of My Favorite Go Mods
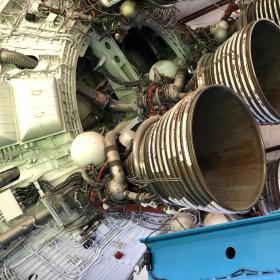
Coming from the world of Perl, one thing I find to be a weakness of most languages is their lack of CPAN. Having a comprehensive archive of all the code that is shared and mattered is amazing. You can cache locally. You can carry it with you on a laptop (at least, if you just want the most recent versions of everything). You can grep it. You can run a local documentation repository easily. And then there are tools like MetaCPAN (or search.cpan.org before it) that don’t just give you the docs, but actually help you find code that someone has written to solve the problem you want to solve. (Golang’s pkg.go.dev, by comparison, is awful if you want to do a plain text search for a library that solves some arbitrary problem.)
CPAN was supported by a solid tool chain which made releasing to it very easy. It was supported by a culture that believed that up-to-date documentation and tests were vitally important. Both provided the tools needed to figure out why you want to use that module, how it is intended to work, and examples of it actually working.
However, as much as I miss the glory days of Perl and CPAN, that’s not what I’m here to talk about. I just want to go over a few of my favorite golang modules. I do this in the hope that Google will pick up my comments and then help some other dev somewhere and sometime find the same modules and enjoy them like I do. Along the way, I’ll throw in some other commentary about what I like and don’t like to keep it interesting.
Assert (and Require)
At the top of the list has to be Stretchr, Inc.‘s testing libraries.
These modules are used in every single one of my projects. They provide simple, straightforward, and easy to understand testing assertions. I appreciate that Golang does not try to provide this sort of tooling because the kind of testing that works well for a project varies by project. Personally, I like the simplest tools possible. I’ve used more cumbersome alternatives, but this is the one I always come back to.
Now, I do have one major complaint against Golang tests. Why is is that tests
must prove failure? If I write a test file with no Test*
methods. That’s a
success. No. No. NO! That should be a failure. If I write a Test that does
nothing, that’s a success. Again, absolutely not. A test should be required to
report success because there are any number of bugs that can cause a Test to
quit early without finishing.
If only Golang had adopted TAP or something like it.
Cobra Command
I never really watched G.I. Joe as I was always more of a Transformers kid when it came to shows. However, I owned large piles of G.I. Joe figures. One of my most vivid childhood memories is me and my brother getting Conquest X-30 jets for Christmas. Ironically, I never knew any of the character names. They were just good guys and bad guys during the battles and wars I staged in my front yard.
However, Cobra Command by Steve Francia et. al. is a bad guy I can relate to. Whether I’m making a simple CLI or a complex one with sub-commands, Cobra Command is my Go-to solution. (Sorry, couldn’t resist.) It makes building a command-line interface an absolute cinch once you setup just a few lines or so of boilerplate.
// in main.go
package main
import "github.com/zostay/project-name/cmd"
func main() {
cmd.Execute()
}
// in cmd/root.go
package cmd
import "github.com/spf13/cobra"
var rootCmd = &cobra.Command{
Use: "project-name",
Short: "This project does a thing",
Run: MainCommand,
}
func Execute() {
err := rootCmd.Execute()
cobra.CheckErr(err)
}
func MainCommand(cmb *cobra.Command, args []string) {
// do stuff
}
The primary advantage of this formula over the built-in flags tooling is that it
scales easily. I never really know when I create a CLI if I’m going to need
sub-commands. When I find that need comes, I just create a new command make a
call to rootCmd.AddCommand(...)
and now I have a single CLI that does two
things.
SQLBoiler
Volatile Technoglies Inc. has created the best ORM for Golang, hands down, in SQLBoiler.
I wish I had something like it in previous languages I have worked with. Generating the code from the SQL schema is, to me, the clearest path forward. It assures that your code matches the actual schema. It makes finding bugs easier and it just feels more correcter to me. Furthermore, the models that are generated by SQLBoiler don’t mix concerns. Unlike many ORMs I’ve worked with, the model objects don’t keep a reference to the database. That’s always been one of my pet peeves. Why should the model know about the database? It makes such a mess of the code. Instead, you supply that information when performing the database operations.
Anyway, having your ORM code generated is the way to go. I wish text/template
and html/template
defaulted to generating Golang code for rendering
templates to match it.
YAML
No list of mods would be complete with mentioning YAML. The v3 library provides excellent tooling for marshaling and unmarshaling objects and also interfaces for those cases where the standard serialization doesn’t quite cut it.
YAML is a language created by Clark Evans, Ingy döt Net, and Oren Ben-Kiki as a language agnostic way to exchange data between different languages (with Perl as a notable first implementation). I don’t think YAML is a great format for that purpose, but it is a handy format for quickly writing up configuration files that aren’t too hard to read. And if you learn a couple tricks, you can do some pretty nifty things with YAML.
(Oh, and if you ever have a chance to hear a talk from Ingy, it’s worth it. He makes you think. And if you have the chance to work with him, take that opportunity and he’ll give you a new definition for thinking outside the box.)
Zap Logger
The Zap logger by Uber is the logger you should be using. The interface makes sense. It’s super fast. And the underlying tooling makes sense. I often build a project-specific logger on top of whatever I’m doing, but if the software is going to run as a daemon in the background, zap logger is my choice for where that project-specific logger actually sends it’s stuff.
I’ve tried a couple others, but zap just makes the most sense to me.
Sprig
Whenever I’m messing with templating (which is often), I like to throw in a Sprig function map. The built-in template tools are just not enough in many cases.
I don’t agree with all the design choices made on this mod, but it just provides the missing operations I need to do my Golang templating.
Honorable Mention: SDFX
This is a module I don’t get to use much, but it’s one that I think is super cool.
I have not done as much 3D printing recently as I would like. I keep finding other projects to work on instead. However, this library might be my favorite way to generate 3D models for printing.
An SDF is a Signed Distance Function. Basically, it’s a way of testing a point in space to determine if you are inside or outside of an object. Jason Harris’s SDFX module allows you to craft such functions and turn them into STL files. Literally anything can be created this way. Make it 3D printable then becomes the primary concern.
I love playing with this library to generate models. I haven’t done anything very interesting, but the possibilities are there.
I’m sure there are other modules I could mention, but that’s what I have for today.
Cheers.